Chat Bot Example
This example shows how to create a Union chat bot for Node.JS using OrbiterMicroNode. To run the chat bot, follow these steps:
- Obtain and install Node.JS from http://nodejs.org/. This sample has been tested up to Node 0.4.8.
- Download the chat bot code from http://www.unionplatform.com/samples/orbitermicronode/chatbot/OrbiterMicroNodeChatBot.zip.
- Unzip the file you downloaded in step 2. A folder named OrbiterMicroNodeChatBot will be created.
- From your system's command prompt, issue the following command from the OrbiterMicroNodeChatBot directory:
node OrbiterMicroNodeChatBot.js tryunion.com 80
When the chat bot runs, you should see a series of updates displayed in the system console similar to those shown in the following diagram:
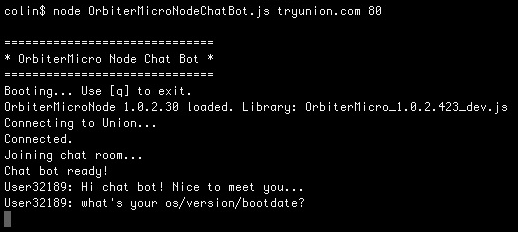
The chat bot connects to the chat room from this OrbiterMicro's chat example, which is runs in a web browser.
Here is the source code for the chat bot:
//============================================================================== // VARIABLES //============================================================================== // The root of the OrbiterMicro object model var net; // A reference to this client's Orbiter object var orbiter; // A reference to this client's MessageManager object var msgManager; // A convenience reference to the UPC object, which lists all available messages var UPC; // The id of the room to create and join var roomID = "chatRoom"; // The frequency with which this client sends automated messages to the room var sendMessageFrequency = 30000; // Command-line arguments var host; var port; var debugMode = false; //============================================================================== // BOOT FUNCTIONS //============================================================================== function main () { // Quit if command line args don't validate var args = process.argv.slice(2); if (args.length < 2) { usage(); return; } out(''); out('============================='); out('* OrbiterMicroNode Chat Bot *'); out('============================='); out('Booting... Use [q] to exit.'); // Parse command-line args host = args[0]; port = args[1]; debugMode = args[2] == "debug"; // Load OrbiterMicroNode module net = require('OrbiterMicroNode_2.0.0.136_Release').net; // Listen for command-line input var stdin = process.openStdin(); stdin.on('data', onStdInData); // Start the client init(); } function usage() { out('Usage:'); out('node OrbiterMicroNodeChatBot.js [host] [port] [debug]'); out(' -debug (optional) enables debug logging') out('E.g. (no debugging):'); out(' node OrbiterMicroNodeChatBot.js tryunion.com 80'); out('E.g. (with debugging):'); out(' node OrbiterMicroNodeChatBot.js tryunion.com 80 debug'); process.exit(); } //============================================================================== // INITIALIZATION //============================================================================== function init () { // Create the Orbiter instance, used to connect to and communicate with Union orbiter = new net.user1.orbiter.Orbiter(); // Register for Orbiter's connection events orbiter.addEventListener(net.user1.orbiter.OrbiterEvent.READY, readyListener, this); orbiter.addEventListener(net.user1.orbiter.OrbiterEvent.CLOSE, closeListener, this); UPC = net.user1.orbiter.UPC; // Register for incoming messages from Union msgManager = orbiter.getMessageManager(); msgManager.addMessageListener(UPC.JOINED_ROOM, joinedRoomListener, this); msgManager.addMessageListener("CHAT_MESSAGE", chatMessageListener, this, [roomID]); // Register for log events if requested if (debugMode) { orbiter.getLog().setLevel("debug"); orbiter.getLog().addEventListener("UPDATE", logUpdateListener); } // Connect to Union orbiter.connect(host, port); out("Connecting to Union..."); } //============================================================================== // ORBITER EVENT LISTENERS //============================================================================== // Triggered when the connection is ready function readyListener (e) { out("Connected."); out("Joining chat room..."); // Create the chat room msgManager.sendUPC(UPC.CREATE_ROOM, roomID); // Join the chat room msgManager.sendUPC(UPC.JOIN_ROOM, roomID); } // Triggered when the connection is closed function closeListener (e) { out("Orbiter connection closed."); } //============================================================================== // CHAT ROOM EVENT LISTENER //============================================================================== // Triggered when a JOINED_ROOM message is received function joinedRoomListener () { out("Chat bot ready!"); // Say hello to the chat room sendMessage("Hello from OrbiterMicroNode! I'm a robot running in Node.JS."); // Send a message every 'sendMessageFrequency' milliseconds var birthtime = new Date(); setInterval(function () { var minutes = Math.floor((new Date() - birthtime)/1000/60); sendMessage("I'm OrbiterMicroNode bot #" + orbiter.getClientID() + "." + " I've been here for " + minutes + " minute(s)."); }, sendMessageFrequency); } //============================================================================== // CHAT SENDING AND RECEIVING //============================================================================== // Sends a chat message to everyone in the chat room function sendMessage (message) { msgManager.sendUPC(UPC.SEND_MESSAGE_TO_ROOMS, "CHAT_MESSAGE", roomID, "true", "", message); } // Triggered when a chat message is received function chatMessageListener (fromClientID, message) { // Show messages from other users if (fromClientID != orbiter.getClientID()) { out("User" + fromClientID + ": " + message); } } //============================================================================== // LOG UPDATE LISTENER //============================================================================== function logUpdateListener (e) { out(e.getLevel() + ": " + e.getMessage()); } //============================================================================== // STD IN/OUT //============================================================================== function out (msg) { console.log(msg); } function onStdInData (data) { var data = data.toString().trim(); var cmd = data.split(':')[0]; var data = data.split(':')[1]; switch (cmd) { case 'exit': case 'quit': case 'q': process.exit(); break; } } //============================================================================== // BOOT THE APP //============================================================================== main();